TMAds JavaScript SDK
TMAds makes it possible for Telegram Mini Apps developers to implement additional monetization via non-intrusive banner ads, interstitials and rewarded videos, and for publishers to reach a constantly growing Mini Apps audience.
Demo
Check out an example Telegram Mini App (Source).
An example of a TMAds banner can be seen below:
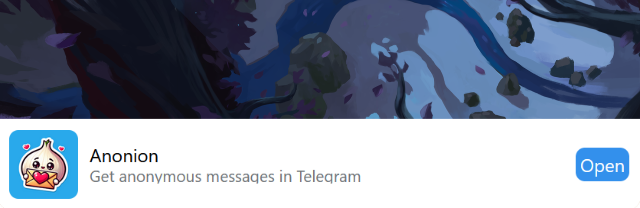
TMAds automatically adjusts banners' appearance to match the Telegram user's app (day/night mode).
Technical requirements
The following browsers/webviews are supported:
- Safari for iOS 12.2+
- Chrome for Android 97+
- Chrome 25+
- Firefox 28+
- iOS Safari 12.2+
- Edge 79+
Usage
Load via CDN (aka include the following line in the <head>
section of your .html file):
<script src="https://cdn.tmads.xyz/tmadsSdk.js"></script>
Make sure that Telegram Mini Apps SDK is loaded, otherwise TMAds might not function properly. Also note that TMAds uses Telegram.WebApp.initData
string to communicate with ad servers.
Initialize with your App Key:
TMAds.init(string app_key);
or
TMAds.init(object app_data);
The latter way should be used when you're using a third-party Telegram SDK wrapper which modifies the original Telegram object structure (e.g. @tma/sdk, which renders Telegram.WebApp
object inaccessible via direct call). The app_data
object should contain the following data:
Name | Type | Description |
---|---|---|
app_key |
string | Required. Your TMAds App Key |
init_data |
string | Required. User data string originally from Telegram.WebApp.initData |
init_data_unsafe |
object | Required. User data object originally from Telegram.WebApp.initDataUnsafe |
debug_mode |
boolean | Optional. If true, enables debug mode which serves test ads immediately without preloading them from TMAds servers. Can be set separately viaTMAds.setDebugMode |
Show banner:
TMAds.show(string position);
position
can be either top
or bottom
(default is bottom
)
Hide banner when needed:
TMAds.hide();
Callbacks
You can add callbacks for successful and failed ad load events via addCallback
function. Additionally, an object with banner_height
field containing the banner's height in pixels and banner_position
field containing the banner's currrent position is passed into onAdLoadSuccess
callback. Since banners are covering the webpage, this information can be used to adjust contents to not be covered by banner.
TMAds.addCallback("onAdLoadSuccess",function({banner_height: int, banner_position: string}){
//ad loaded and banner displayed
});
TMAds.addCallback("onAdLoadFail",function(){
//ad failed to load and display (no fill, network issues etc)
});
To remove a previously added callback, use removeCallback
:
TMAds.removeCallback(string callback_name);
Interstitials and Rewarded videos
You can use TMAds to display interstitial and rewarded video ads as well:
TMAds.checkInterstitialAd()
.then(()=>{
//interstitial ad preloaded
})
.catch(()=>{
//interstitial ad failed to preload (no fill, network issues etc)
});
TMAds.showInterstitialAd()
.then(()=>{
//interstitial ad has been successfully shown and closed
})
.catch(()=>{
//interstitial ad failed to show (likely due to the network issues)
});
TMAds.checkRewardedAd()
.then(()=>{
//rewarded ad preloaded
})
.catch(()=>{
//rewarded ad failed to preload (no fill, network issues etc)
});
TMAds.showRewardedAd()
.then(()=>{
//rewarded ad has been successfully shown and closed, user should be rewarded
})
.catch(()=>{
//user decided to skip the rewarded ad, shouldn't be rewarded
});
It's a good practice to preload interstitals and rewarded ads before showing. Otherwise, TMAds will attempt to preload an ad, and in case of preload failure promise returned by the showing function will be rejected.
Additionally, the following callbacks for interstitials and rewarded ads are available:
TMAds.addCallback("onInterstitialAdLoadSuccess",function(){
//interstitial ad preloaded
});
TMAds.addCallback("onInterstitialAdLoadFail",function(){
//interstitial ad failed to preload (no fill, network issues etc)
});
TMAds.addCallback("onInterstitialAdShowSuccess",function(){
//interstitial ad has been successfully shown and closed
});
TMAds.addCallback("onInterstitialAdShowFail",function(){
//interstitial ad failed to show (likely due to the network issues)
});
TMAds.addCallback("onRewardedAdLoadSuccess",function(){
//rewarded ad preloaded
});
TMAds.addCallback("onRewardedAdLoadFail",function(){
//rewarded ad failed to preload (no fill, network issues etc)
});
TMAds.addCallback("onRewardedAdShowSuccess",function(){
//rewarded ad has been successfully shown and closed, user should be rewarded
});
TMAds.addCallback("onRewardedAdShowFail",function(){
//user decided to skip the rewarded ad, shouldn't be rewarded
});
Several guidelines to follow:
- Show interstitial and rewarded videos only after user gesture (e.g. a tap), otherwise video playback will be disabled on mobile devices due to browsers' policies. A notable exception are static interstitial ads (see below).
- On Android the Mini App always expands to its' maximum height when an interstitial or rewarded ad is shown. It might not be the case on iOS, so it's better to manually call
Telegram.WebApp.expand();
when showing a fullscreen ad. - Also, you should manually call Telegram's expand method if you're using a third-party Telegram SDK wrapper.
Static interstitials (SDK version 1.0.5+)
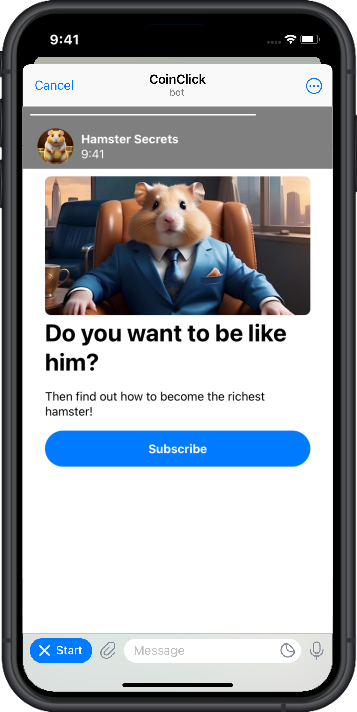
Starting from SDK version 1.0.5, a static interstitial format can be displayed alongside with video-based ads. By default it's up to TMAds to decide which format to load. However, you can opt-in to display only static interstitial by passing true
boolean value as an argument to the following functions:
TMAds.checkInterstitialAd
TMAds.checkRewardedAd
TMAds.showInterstitialAd
TMAds.showInterstitialAd
An example:
TMAds.checkInterstitialAd(true); //will try to preload only static interstitial ads
Please note that show
functions will only take that argument into account if a corresponding check
function has not been called before — otherwise it will display an already preloaded ad regardless of its' format.
Unlike video ads, static interstitials can be displayed without prompting user gesture.
Rewarded surveys
Another TMAds format is a rewarded survey. You can preload and show these as following:
TMAds.checkRewardedSurvey()
.then(()=>{
//rewarded survey preloaded
})
.catch(()=>{
//rewarded survey failed to preload (no fill, network issues etc)
});
TMAds.showRewardedSurvey()
.then(()=>{
//user has completed the survey, should be rewarded
})
.catch(()=>{
//user decided to skip the survey, shouldn't be rewarded
});
Additionally, the following callbacks for rewarded surveys are available:
TMAds.addCallback("onRewardedSurveyLoadSuccess",function(){
//rewarded survey preloaded
});
TMAds.addCallback("onRewardedSurveyFail",function(){
//rewarded survey failed to preload (no fill, network issues etc)
});
TMAds.addCallback("onRewardedSurveySuccess",function(){
//user has completed the survey, should be rewarded
});
TMAds.addCallback("onRewardedSurveyFail",function(){
//user decided to skip the survey, shouldn't be rewarded
});
Inline rewarded ads
TMAds can be implemented into bots without a Mini App. Rewarded videos and surveys are supported.
Send the following link(s) via KeyboardButton:
To display a rewarded video or a survey (will be picked during opening a TMAds window):
https://inline.tmads.xyz/YOUR_TMADS_APP_KEY/USER_TELEGRAM_ID/USER_LANGUAGE_CODE
To display a rewarded video:
https://inline.tmads.xyz/YOUR_TMADS_APP_KEY/USER_TELEGRAM_ID/USER_LANGUAGE_CODE/video
To display a survey:
https://inline.tmads.xyz/YOUR_TMADS_APP_KEY/USER_TELEGRAM_ID/USER_LANGUAGE_CODE/survey
USER_TELEGRAM_ID
and USER_LANGUAGE_CODE
are values of id
and language_code
fields of a User object passed in a from
field of a Message object of an update sent to the bot.
Upon completion of an ad or a survey, a service message will be sent to your bot, with web_app_data
containing TMAdsVideoEvent
or TMAdsSurveyEvent
respectively.
Example of a link:
https://inline.tmads.xyz/5e56a0575c837bd04f06e50fe501779c/12345678/en/video
Theming
You can set banner's color theme via setTheme
function in order to make it look more native to your Mini App:
TMAds.setTheme(object theme_obj);
theme_obj
is an object containing theme colors. Supported values are:
Name | Description | Default value |
---|---|---|
bg_color |
Background color of the banner | var(--tg-theme-bg-color) |
text_color |
Main text color | var(--tg-theme-text-color) |
secondary_color |
Secondary text color | var(--tg-theme-hint-color) |
button_color |
Button color | var(--tg-theme-button-color) |
button_text_color |
Button text color | var(--tg-theme-button-text-color) |
#rrggbb
, rgb()
or any other CSS-supported format, including referencing already defined CSS variables via var()
.Check Telegram's ThemeParams documentation to get more info on default colors.
Debug mode
Debug mode allows TMAds to immediately display test ads without preloading them from servers. You can enable it by calling the following function before calling TMAds.init
:
TMAds.setDebugMode(bool debug_mode);
Make sure to switch debug mode off in production builds in order to display real ads.
Passing additional user data
You can pass additional user data via setUserData
function:
TMAds.setUserData(object user_data);
user_data
is an object containing various user data. Currently, the following data is supported:
Name | Description |
---|---|
age |
User's age, in full years |
yob |
User's year of birth (4 digits) |
gender |
User's gender, M for male, F for female |
Where can I get an App Key?
Enable debug mode or use 5e56a0575c837bd04f06e50fe501779c
as an App Key for testing purposes. Demo ads will be displayed.
In order to get your own App Key:
- Register in our Publisher dashboard
- Navigate to "Apps" page (if you're not here)
- Press "Add new app" button
- Fill out necessary information about your app (name, Telegram bot handle, category) and press "Create"
- You will be redirected to your newly created app's page. App Key is displayed right in the "App settings" section
- After you've done integrating TMAds in your app, got to the app's page and press "Submit for review"
- Once your app is approved, it will start displaying live ads. Until that, demo ads will be served